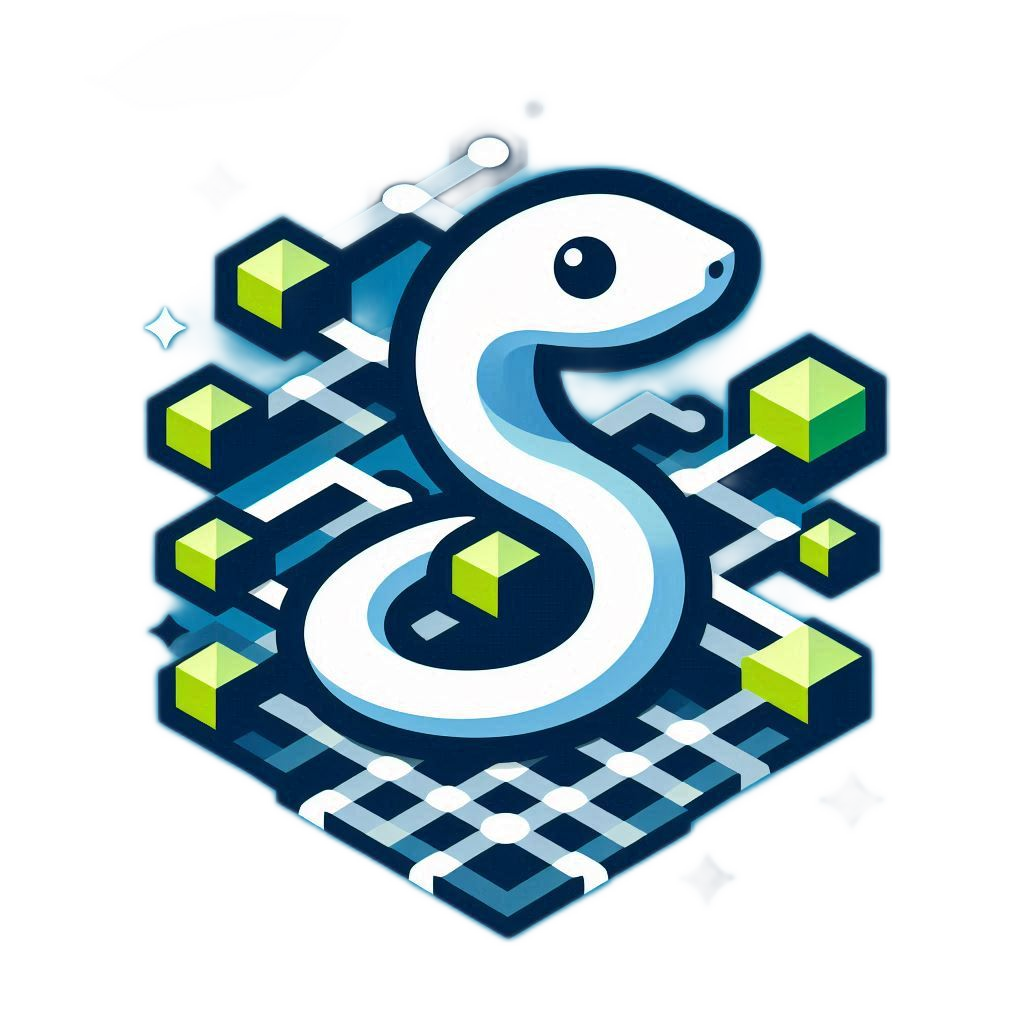
Section Navigation
Getting Quick Started
This guide covers some basic usage patterns and best practices to help you get started with Traversecraft.
Simple GridWorld Example
Here is a simple example of how to create a grid world and an agent.
from traverseCraft.world import CreateGridWorld
from traverseCraft.agent import GridAgent
Let's create a grid world and construct it.
gridWorld = CreateGridWorld(worldName="Simple Grid World", cols=10, rows=10, cellSize=36)
gridWorld.constructWorld()
gridWorld.setBlockPath(blockCells=[[1,1],[2,2],[3,3],[4,3]])
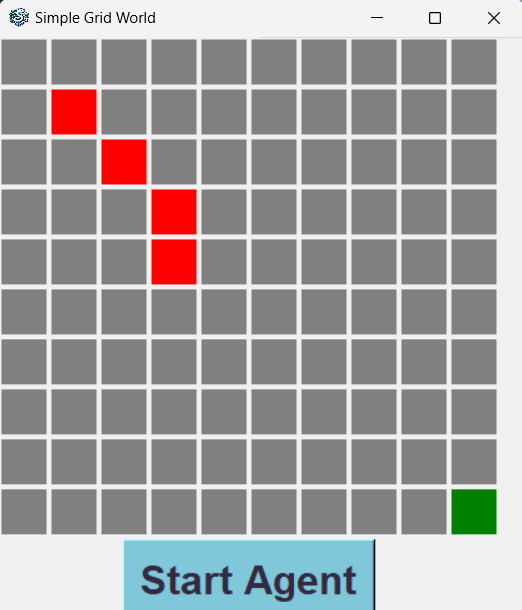
Now, let's create an agent and place it in the grid world!
gridAgent = GridAgent(agentName="Simple Grid Agent", world=gridWorld, agentPos=[0,0])
gridWorld.setAgent(agent=gridAgent)
Now, we apply the DFS algorithm (Depth-First-Search) to find the shortest path to the goal.
def dfs(agent, i, j, visited):
if(agent.checkGoalState(i, j)):
return True
if(visited[i][j]):
return False
visited[i][j] = True
# move up
if(i > 0 and not visited[i-1][j] and agent.moveAgent(i-1, j)):
if(dfs(agent, i-1, j, visited)):
return True
agent.moveAgent(i, j)
# move down
if(i < 4 and not visited[i+1][j] and agent.moveAgent(i+1, j)):
if(dfs(agent, i+1, j, visited)):
return True
agent.moveAgent(i, j)
# move left
if(j > 0 and not visited[i][j-1] and agent.moveAgent(i, j-1)):
if(dfs(agent, i, j-1, visited)):
return True
agent.moveAgent(i, j)
# move right
if(j < 4 and not visited[i][j+1] and agent.moveAgent(i, j+1)):
if(dfs(agent, i, j+1, visited)):
return True
agent.moveAgent(i, j)
return False
Let's define a callback function that our agent will use.
def toDo():
visited = [[False for _ in range(5)] for _ in range(5)]
print("Agent Started moving around the world!")
if(dfs(gridAgent, 0, 0, visited)):
print("Agent reached the goal state!")
else:
print("Agent could not reach the goal state!")
Let's set the callback function and start the simulation!
gridAgent.setAlgorithmCallBack(toDo)
gridWorld.showWorld()
For more information, you can print the summary of the agent as well as the world!
print(gridWorld.summary())
print(gridAgent.summary())
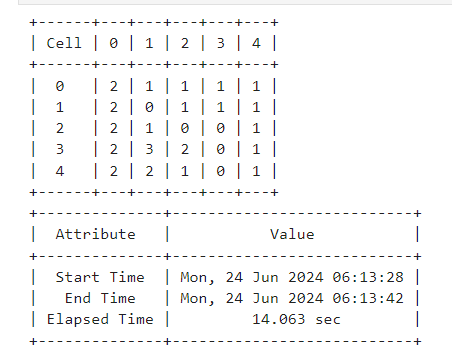
Simple TreeWorld Example
Here is a simple example of how to create a tree world and an agent.
from traverseCraft.world import CreateTreeWorld
from traverseCraft.agent import TreeAgent
treeWorldInfo = {
'adj': {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F', 'G'],
'D': [],
'E': [],
'F': [],
'G': []
},
'position': {
'A': (300, 100),
'B': (150, 200),
'C': (450, 200),
'D': (100, 300),
'E': (200, 300),
'F': (300, 300),
'G': (400, 400)
},
'root': 'A',
'goals': ['G']
}
Let's create a tree world and construct it.
treeWorld = CreateTreeWorld(worldName="Simple Tree World", worldInfo=treeWorldInfo)
treeWorld.constructWorld()
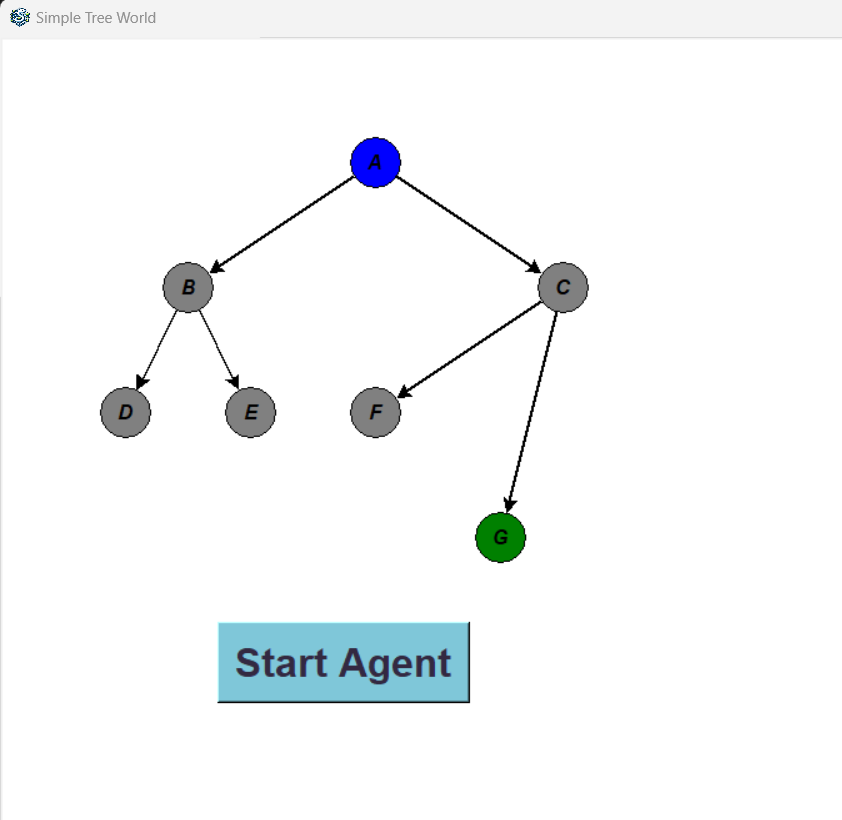
Now, let's create an agent and place it in the tree world!
treeAgent = TreeAgent(agentName="Simple Tree Agent", world=treeWorld)
treeWorld.setAgent(agent=treeAgent)
Now, let's apply the DFS algorithm (Depth-First Search) to find the shortest path to the goal.
def dfs(nodeId, agent):
if agent.checkGoalState(nodeId):
return True
for child in treeWorld.nodeMap[nodeId].children:
agent.moveAgent(child.id)
if dfs(child.id, agent):
return True
agent.moveAgent(nodeId)
return False
Let's define a callback function that our agent will use.
def toDo():
print("Agent Started moving around the world!")
if(dfs(treeWorld.root.id, treeAgent)):
print("Agent reached the goal state!")
else:
print("Agent could not reach the goal state!")
Let's set the callback function and start the simulation!
treeAgent.setAlgorithmCallBack(toDo)
treeAgent.showWorld()
For more information, you can print the summary of the agent as well as the world!
print(TreeWorld.summary())
print(TreeAgent.summary())
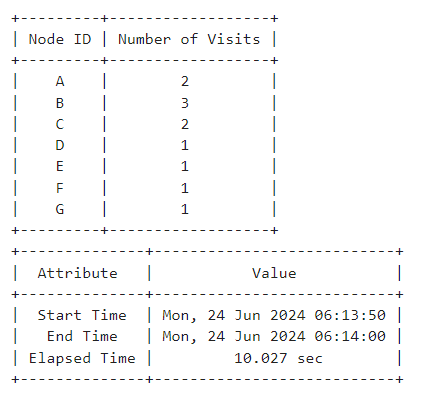
Simple GraphWorld Example
Here is a simple example of how to create a graph world and an agent.
from traverseCraft.world import CreateGraphWorld
from traverseCraft.agent import GraphAgent
graphWorldInfo = {
'adj': {
'A': ['B', 'C'],
'B': ['C', 'D', 'E'],
'C': ['B', 'F'],
'D': ['E'],
'E': ['F', 'G'],
'F': ['E', 'G'],
'G': []
},
'position': {
'A': (100, 200),
'B': (200, 100),
'C': (200, 300),
'D': (300, 200),
'E': (400, 100),
'F': (400, 300),
'G': (500, 200)
},
'goals': ['G']
}
Let's create a graph world and construct it.
graphWorld = CreateGraphWorld(worldName="Simple Graph World", worldInfo=graphWorldInfo)
graphWorld.constructWorld()
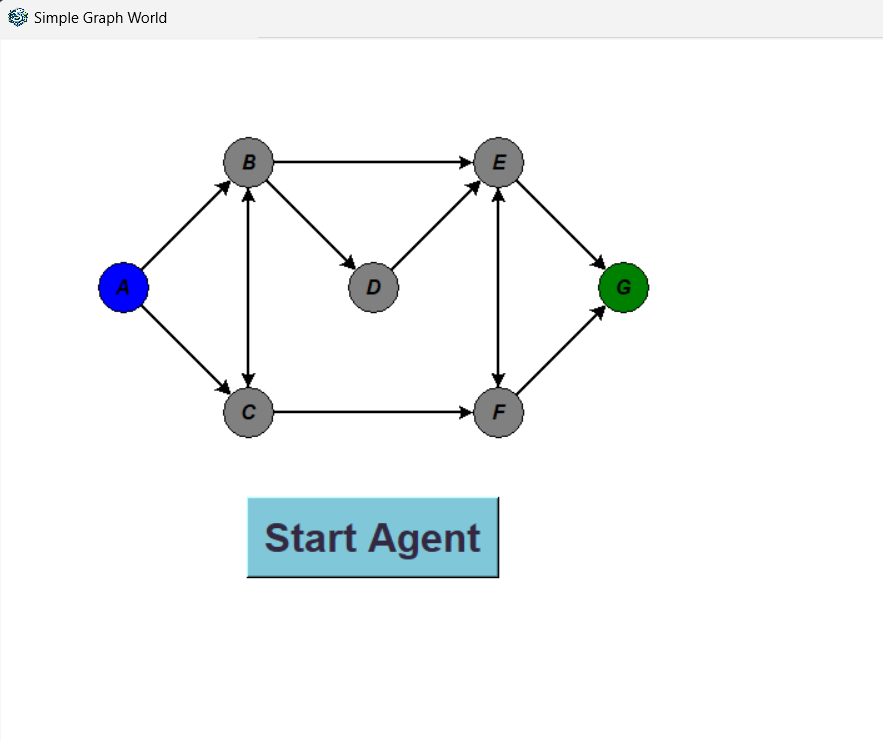
Now, let's create an agent and place it in the graph world!
graphAgent = GraphAgent(agentName="Simple Graph Agent", world=graphWorld, startNodeId='A')
graphWorld.setAgent(agent=graphAgent)
Now, let's apply the DFS algorithm (Depth-First Search) to find the shortest path to the goal.
def dfs(nodeId, agent, visited=[]):
if agent.checkGoalState(nodeId):
return True
visited.append(nodeId)
for neighbor in graphWorld.nodeMap[nodeId].neighbors:
if neighbor.id in visited:
continue
agent.moveAgent(neighbor.id)
if dfs(neighbor.id, agent, visited):
return True
agent.moveAgent(nodeId)
return False
Let's define a callback function that our agent will use.
def toDo():
print("Agent Started moving around the world!")
if(dfs('C', graphAgent)):
print("Agent reached the goal state!")
else:
print("Agent could not reach the goal state!")
Let's set the callback function and start the simulation!
graphAgent.setAlgorithmCallBack(toDo)
graphAgent.showWorld()
For more information, you can print the summary of the agent as well as the world!
print(graphWorld.summary())
print(graphAgent.summary())
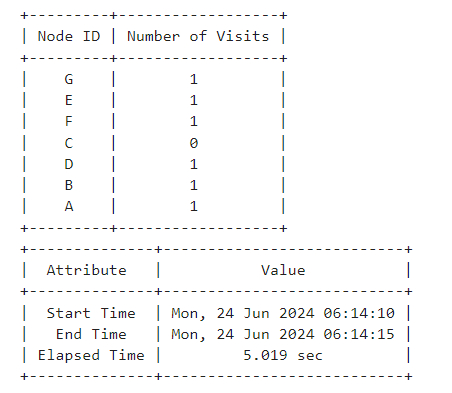